3 min read
Monitoring Twitch Streams with a Stream Deck and Cloudflare Workers
I found myself wanting to see when my favorite Twitch creators went live from my Elgato Stream Deck (mostly because I can't stand push notifications). I could have used an existing plugin, but what fun is that? Naturally, I set off to build my own using Cloudflare Workers.
Design
The goal was simple: show a black and white version of the creator's profile image when they're offline, and a color image with a 'Live' icon when they're online. That way, I can quickly see which creators are online at any time.
Twitch API Auth
The first thing we need to do is create a client to talk to the Twitch API. This involves registering an application with Twitch, and automating token refresh so that we can make server-side API calls from Cloudflare Workers.
Only one token can be used at a time and lasts about 60 days before it needs refreshing, so we store the token in Durable Object Storage, and automatically refresh when it expires:
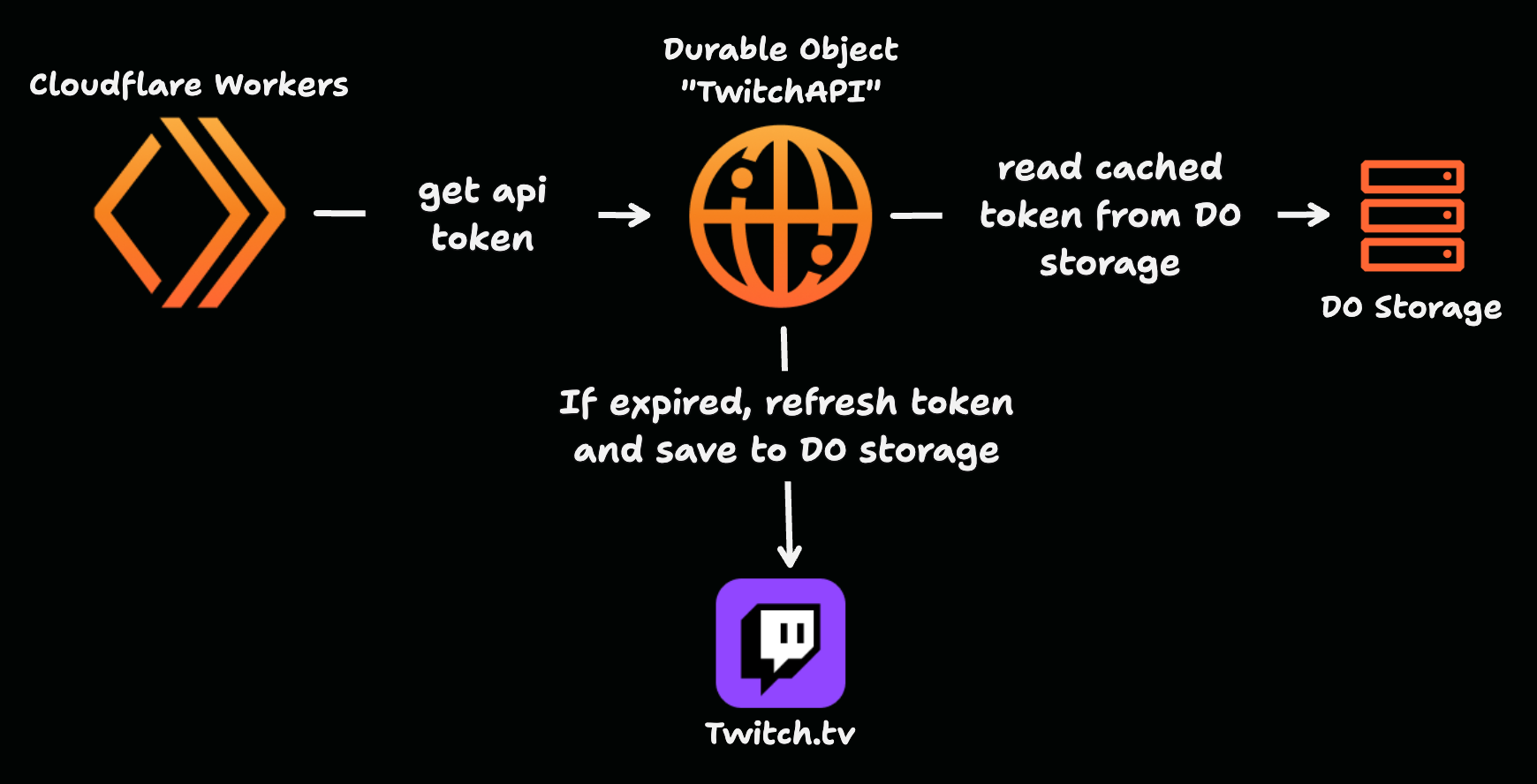
Check Creator Status
Now that we have a token, we need to fetch the creator to get their profile image and live status so that we can determine which image to serve. To do this, we will use our newly created API token to call the Twitch API to check the creator's live status:
const honoApp = new Hono<HonoApp>().get(
'/api/:creator/streamdeck',
zValidator('param', z.object({ creator: z.string() })),
async (c) => {
const { creator } = c.req.valid('param')
const twitch = getTwitchAPIClient(c.env)
const res = await twitch.getUser(creator)
if (res.data.length == 0) {
return c.notFound()
}
const profileImageUrl = res.data[0].profile_image_url
// check live status
const res2 = await twitch.getChannelStatus(creator)
const stream = res2.data.find((s) => s.type === 'live')
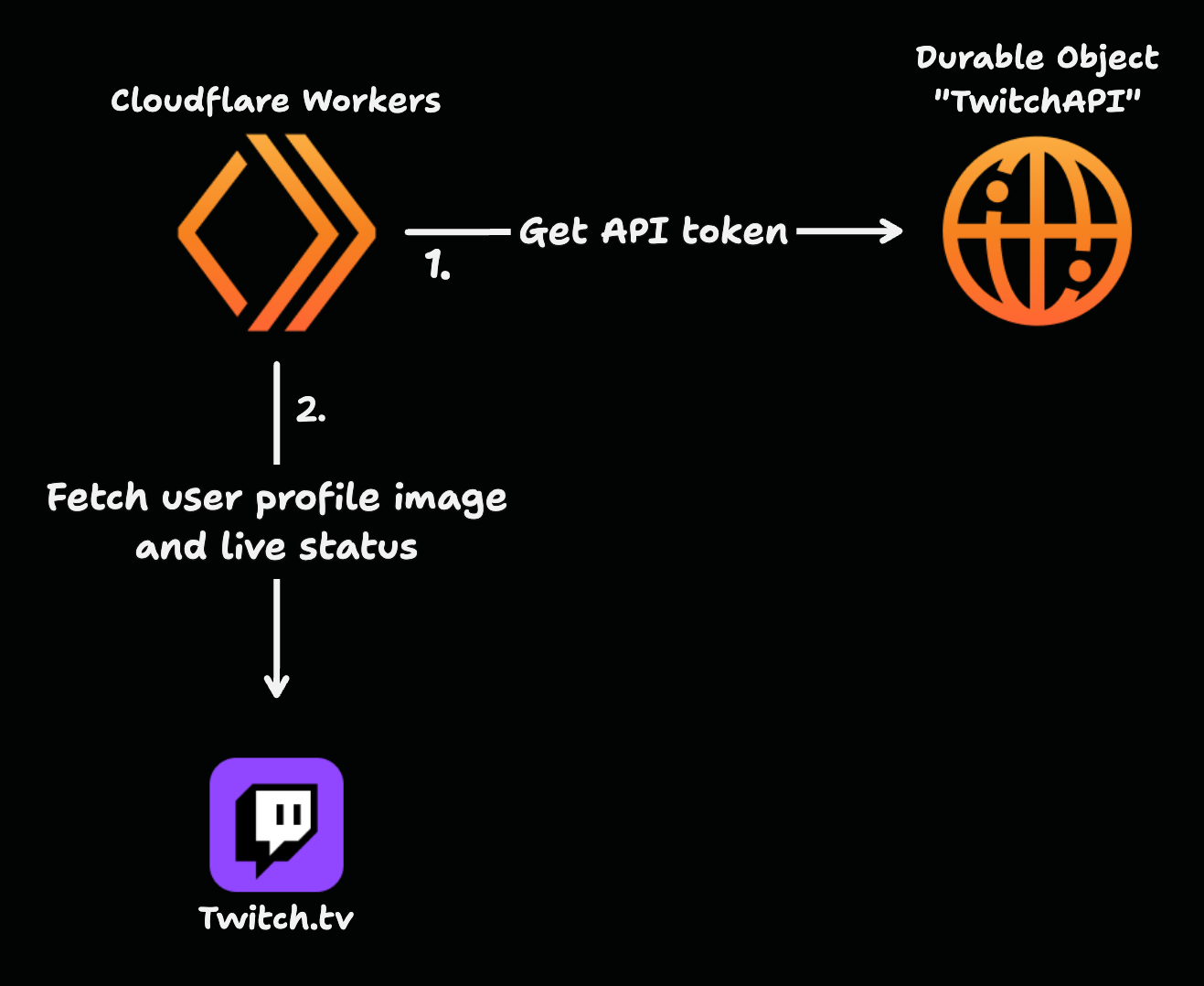
Transform Profile Image Using Cloudflare Images
Finally, we need to transform it to be 280x280 pixels (the optimal size for Stream Deck buttons), and apply effects based on the creator's status. Cloudflare Images makes this incredibly easy with the new Images Binding:
// fetch images
const [profileImageRes, liveIconRes] = await Promise.all([
fetch(profileImageUrl),
c.env.ASSETS.fetch('http://assets/live.png'),
])
const profileImage = profileImageRes.body
const liveIcon = liveIconRes.body
if (!profileImageRes.ok || profileImage === null) {
throw new Error('unable to fetch profileImage')
}
if (!liveIconRes.ok || liveIcon === null) {
throw new Error('unable to fetch liveIcon')
}
if (stream) {
return (
await c.env.IMAGES.input(profileImage)
.transform({
fit: 'scale-down',
width: 280,
})
.draw(
c.env.IMAGES.input(liveIcon).transform({
width: 120,
height: 60,
}),
{ bottom: 5, left: 80, opacity: 100 }
)
.output({
format: 'image/png',
})
).response()
} else {
return (
await c.env.IMAGES.input(profileImage)
.transform({
fit: 'scale-down',
width: 280,
saturation: 0,
})
.output({
format: 'image/png',
})
).response()
}
The full code for this API endpoint is available here.
When a user is online, we crop the image and overlay a Live icon (which is served from Workers Assets). Otherwise, we crop the image and set the saturation to 0 to make the image black and white. The new Images binding makes this so easy, I love it!
Configuring the Stream Deck
Using the API Ninja plugin, we can configure a button to fetch the endpoint every minute so that we always know whether a creator is live:
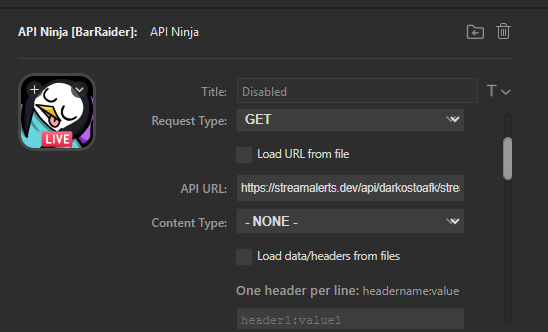
Summary
Now, in addition to allergens and the weather, I can see when my favorite Twitch creators are live using my Stream Deck!
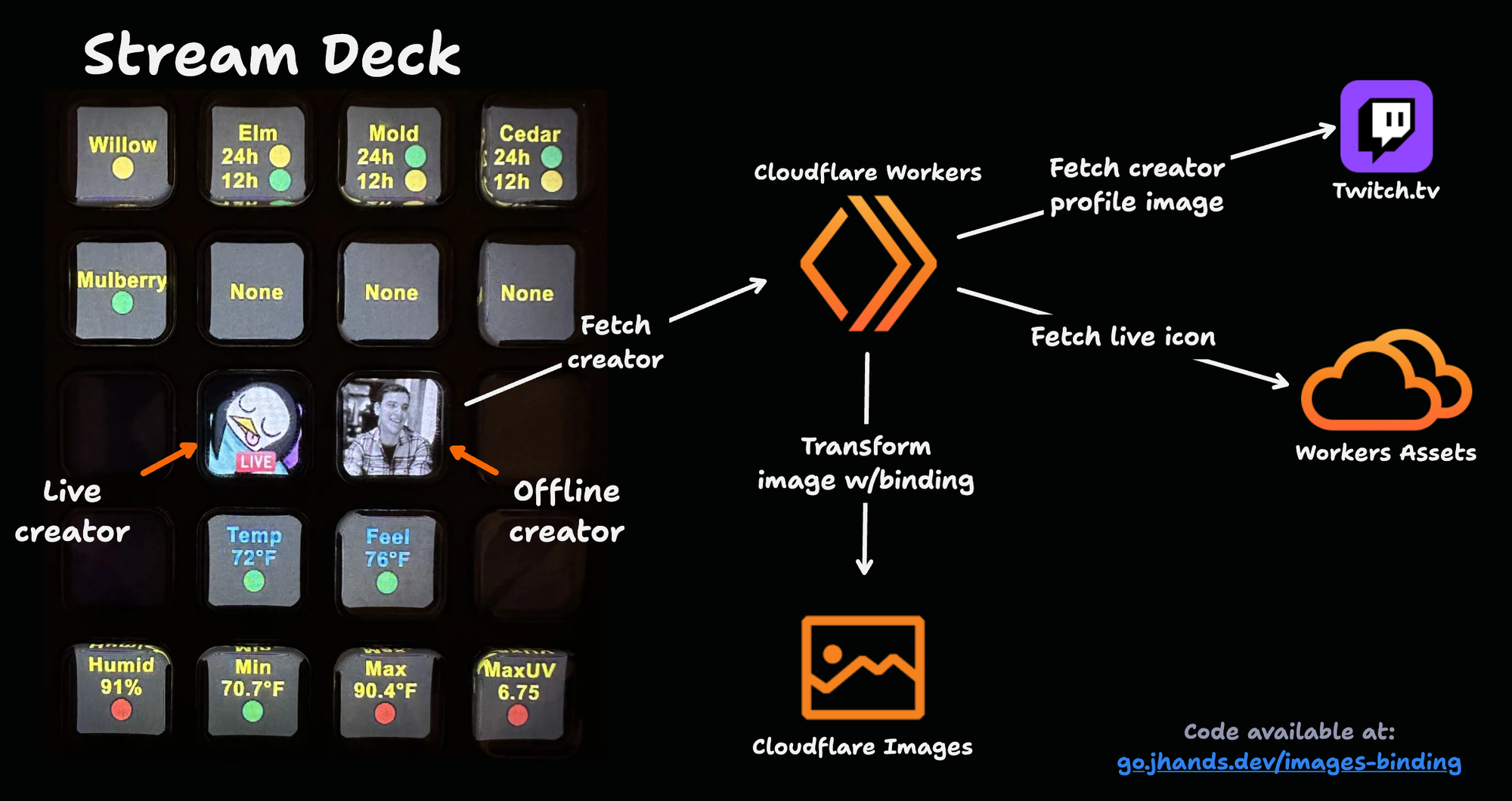